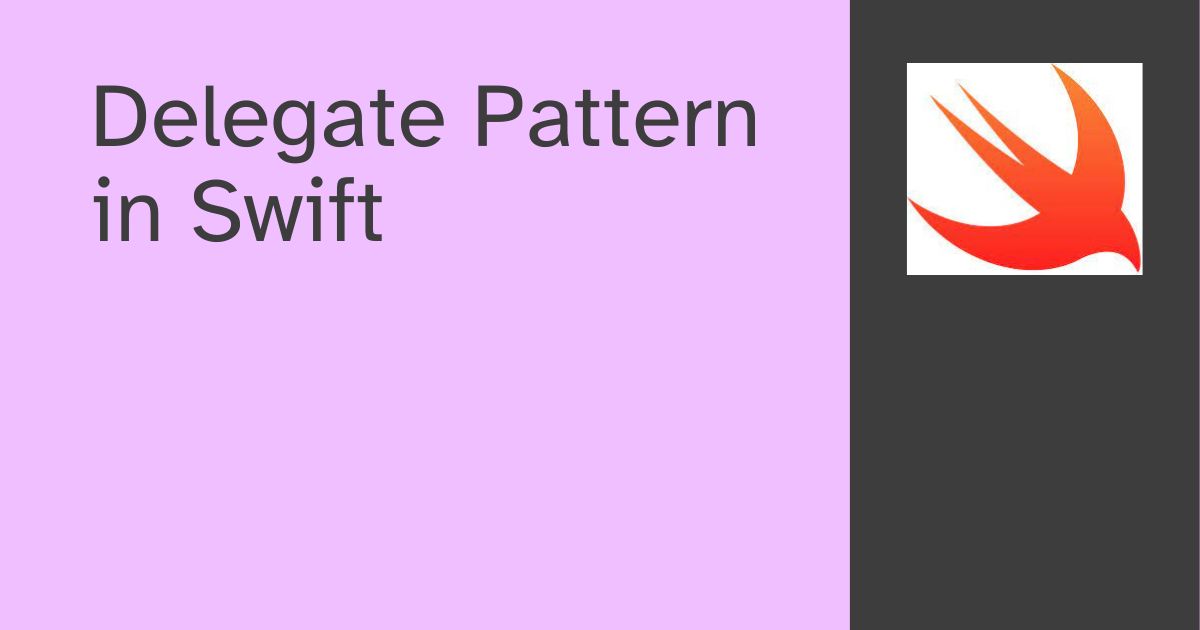
Delegate Pattern in Swift
The delegate pattern in Swift is a design pattern that enables one object to act on behalf of another. It is commonly used to establish communication between two objects, where one object (the delegate) delegates certain responsibilities or actions to another object.
Here’s a step-by-step explanation of how the delegation pattern works and how you can implement it in Swift:
Step 1 – Define a Protocol:
Start by defining a protocol that declares the methods or properties that the delegate should implement. This protocol acts as a contract, ensuring that any class conforming to it provides the necessary functionality.
protocol MyDelegate: AnyObject { func didSomething() }
The AnyObject
keyword is used to specify that only class types can conform to this protocol, making it suitable for delegation.
Step 2 – Create a Delegate Property:
In the class that needs to delegate certain tasks, create a property to hold the delegate. This property should be weak to prevent retain cycles.
class MyClass { weak var delegate: MyDelegate? func performAction() { // Do some work // Notify the delegate delegate?.didSomething() } }
Step 3 – Conform to the Protocol:
Any class that wants to act as a delegate must conform to the defined protocol. Implement the required methods or properties from the protocol.
class MyDelegateImplementation: MyDelegate { func didSomething() { print("Delegate did something!") } }
Step 4 – Set the Delegate:
Instantiate the delegate object and set it as the delegate for the class that needs to delegate tasks.
let myObject = MyClass() let delegateImplementation = MyDelegateImplementation() myObject.delegate = delegateImplementation
Step 5 – Invoke Delegate Methods:
When the class needs to delegate a task, it calls the methods or properties defined in the protocol on its delegate.
myObject.performAction() // This will trigger the delegate's didSomething method
In this example, when performAction
is called on myObject
, it checks if a delegate is set and calls the didSomething
method on the delegate if it exists.
This pattern is powerful because it allows for loose coupling between objects. The object that delegates tasks doesn’t need to know the specifics of the object handling those tasks; it only relies on the protocol. This promotes a modular and maintainable codebase.
Read More:
delegate
Top iOS Interview Questions and Answers